1. 이미지를 확대 또는 축소시키는 resize 코드 예제
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
import cv2
source = cv2.imread('data/images/sample.jpg',1)
print(source.shape) # 이미지 확대 축소
# 1은 100% , 0.6은 60% , 1.8은 180% 확대 / 축소 가능
scaleX = 0.6
scaleY = 0.6
scaleDown = cv2.resize(source,None, fx=scaleX, fy=scaleY, interpolation=cv2.INTER_LINEAR)
print(scaleDown.shape) ## 확대
scaleX = 1.8
scaleY = 1.8
scaleUp = cv2.resize(source, None, fx=scaleX, fy=scaleY, interpolation=cv2.INTER_LINEAR)
print(scaleUp.shape) cv2.imshow('original', source)
cv2.imshow('scaleDown',scaleDown )
cv2.imshow('scaleUp', scaleUp)
cv2.waitKey(0)
cv2.destroyAllWindow()
|
cs |
** 상기 코드 print 출력값
print(scaleDown.shape) - > ( 512, 512, 3 )
print(scaleDown.shape) - > ( 307, 307, 3 )
print(scaleUp.shape) -> ( 922, 922, 3 )
# 이미지 확대, 축소의 parameters
cv2.resize ( img , dsize, fx, fy, interpolation )
- img : image file
- dsize : 가로, 세로 형태의 튜플을 지정하여, 확대 및 축소 가능 ex) (100, 200)
- fx : 가로 사이즈의 배수 , 2배 확대는 2.0 , 1/2 축소는 0.5 ex
- fy : 세로 사이즈의 배수
- interpolation : 보간법 ( 어떤 방식으로 이미지 값을 채울것인가 )
INTER_NEAREST
INTER_LINEAR
INTER_AREAINTER_CUBIC
INTER_LANCZOS4
** 위의 코드와 같이 fx, fy 를 활용하여 비율로 조절해도 되고 ,
cv2.resize(source, dsize(300,300), interpolation=cv2.INTER_LINEAR )
으로 , dsize를 지정해서 변경하여도 상관없다.
2. 이미지를 Crop 하는 코드 예제
Crop 은 원본 이미지에서 원하는 부분만 잘라서 가져오는 것을 말한다.
1
2
3
4
5
6
7
8
9
10
11
12
|
import cv2
source = cv2.imread('data/images/sample.jpg',1)
crop_img = source[ 100:200 , 150 :250]
# x축의 150 : 250 까지
# y축의 100 : 200 까지만 잘라온다.
cv2.imshow('Cropped Img', crop_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
|
cs |
위의 코드 결과는
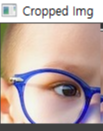
로 print(crop_img.shape)의 값은 ( 100, 100, 3 ) 이다.
'IT 프로그래밍 관련 > OpenCV' 카테고리의 다른 글
OpenCV threshold, 이미지형태 변환(dilate,erode, opening, closing) (0) | 2021.04.22 |
---|---|
OpenCV 비디오파일 열기, 내 캠으로 비디오파일 저장 (0) | 2021.04.21 |
OpenCV Image에 선 그리기 (ImageDraw) (0) | 2021.04.20 |
OpenCV 이미지 열기, 화면 표시 방법, 이미지 저장 (0) | 2021.04.20 |
python에서의 OpenCV 활용 (0) | 2021.04.20 |
댓글